«Tkinter « Projects in Tkinter
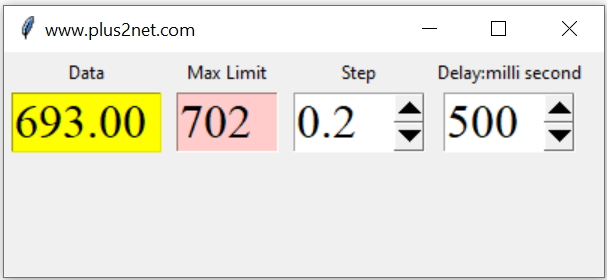
Displaying incremental data based on timer with step value & upper limit in Entry widget of Tkinter
Column | Details |
Data | Displays the final reading after the calculations |
Max Limit | Upper limit for Data column value, if increased then the Data value is allowed to change. |
Step | Spinbox, the increment value for Data. |
Delay | Delay in milli-seconds. Time for updating the readings using the step value |
import tkinter as tk
from tkinter import *
my_w = tk.Tk()
my_w.geometry("400x150") # Size of the window
my_w.title("www.plus2net.com") # Adding a title
font1=('Times 24 normal')
l1=tk.Label(my_w,text='Data')
l1.grid(row=0,column=0,pady=3)
l2=tk.Label(my_w,text='Max Limit')
l2.grid(row=0,column=1,pady=3)
l3=tk.Label(my_w,text='Step')
l3.grid(row=0,column=2,pady=3)
l4=tk.Label(my_w,text='Delay:milli second')
l4.grid(row=0,column=3,pady=3)
e1_d=tk.DoubleVar(value=688.4)
e1 = tk.Entry(my_w, width=6,bg='yellow',
textvariable=e1_d,font=font1) # added one Entry box
e1.grid(row=1,column=0,padx=5)
e_max_i=tk.IntVar(value=702)
e_max = tk.Entry(my_w, width=4,bg='#FFCCCB',
textvariable=e_max_i,font=font1) # added one Entry box
e_max.grid(row=1,column=1,padx=5)
sb1_d=tk.DoubleVar(value=0.2)
sb1 = tk.Spinbox(my_w, from_= 0.2, to = 3.0,width=4, increment=0.2,
textvariable=sb1_d,font=font1)
sb1.grid(row=1,column=2,padx=5)
sb2_i=tk.IntVar(value=500)
sb2 = tk.Spinbox(my_w, from_= 500, to = 5000,width=4, increment=500,
textvariable=sb2_i,font=font1)
sb2.grid(row=1,column=3,padx=5)
def my_upd(*args):
i=e1_d.get()+sb1_d.get()
if(i<=e_max_i.get()): # check upper limit
i="{:.2f}". format(i) # formating upto 2 decimal place
e1_d.set(i)
my_w.after(sb2_i.get(),my_upd)
my_upd() # start
e_max_i.trace('w',my_upd) # if the max limit is changed
my_w.mainloop() # Keep the window open
« Entry
« Python Tkinter Projects
«Spinbox « DoubleVar
← Subscribe to our YouTube Channel here