
Tkinter text zoom in and out using buttons and using menus and using scale to trigger the font size
import tkinter as tk
my_w = tk.Tk()
my_w.geometry("400x300")
l1 = tk.Label(my_w,text='Your Name', width=10) #added one Label
l1.grid(row=1,column=1)
font1=['times',12,'normal']
t1 = tk.Text(my_w,width=10,height=1,font=font1) #text box
t1.grid(row=1,column=2,columnspan=2,pady=10)
def my_fun(str1):
if(str1=='plus'):
font1[1]=font1[1]+2
else:
font1[1]=font1[1]-2
t1.config(font=font1)
b1=tk.Button(my_w,text='+',command=lambda:my_fun('plus'))
b1.grid(row=2,column=2)
b2=tk.Button(my_w,text='-',command=lambda:my_fun('minus'))
b2.grid(row=2,column=3)
my_w.mainloop()
Using menubutton

More about Menu buttons
import tkinter as tk
my_w = tk.Tk()
my_w.geometry("400x300")
l1 = tk.Label(my_w,text='Your Name', width=10) #added one Label
l1.grid(row=1,column=1)
font1=['times',12,'normal']
t1 = tk.Text(my_w,width=10,height=1,font=font1) #text box
t1.grid(row=1,column=2,columnspan=2,pady=10)
def my_fun(str1):
if(str1=='plus'):
font1[1]=font1[1]+2
else:
font1[1]=font1[1]-2
t1.config(font=font1)
mb=tk.Menubutton(my_w,text='My Menu',
activebackground='lightyellow',font=font1,
underline=4,relief='raised',width=20)
mb.grid(row=2,column=1,padx=10)
mb.menu=tk.Menu(mb)
mb['menu']=mb.menu
mb.menu.add_command(label='Zoom ++',command=lambda:my_fun('plus'))
mb.menu.add_command(label='Zoom --',command=lambda:my_fun('minus'))
b1=tk.Button(my_w,text='+',command=lambda:my_fun('plus'))
b1.grid(row=2,column=2)
b2=tk.Button(my_w,text='-',command=lambda:my_fun('minus'))
b2.grid(row=2,column=3)
my_w.mainloop()
Using scale
More about scale here
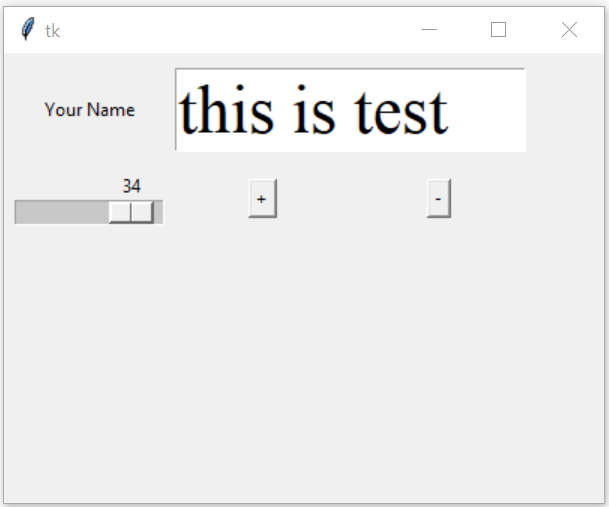
import tkinter as tk
my_w = tk.Tk()
my_w.geometry("400x300")
l1 = tk.Label(my_w,text='Your Name', width=10) #added one Label
l1.grid(row=1,column=1)
font1=['times',12,'normal']
t1 = tk.Text(my_w,width=10,height=1,font=font1) #text box
t1.grid(row=1,column=2,columnspan=2,pady=10)
def my_fun(str1):
if(str1=='plus'):
font1[1]=font1[1]+2
else:
font1[1]=font1[1]-2
t1.config(font=font1)
def my_fun2(value):
font1[1]=my_scale1.get()
t1.config(font=font1)
my_scale1 = tk.Scale(my_w, from_=12, to=26, orient='horizontal',command=my_fun2)
my_scale1.grid(row=2,column=1,padx=4)
b1=tk.Button(my_w,text='+',command=lambda:my_fun('plus'))
b1.grid(row=2,column=2)
b2=tk.Button(my_w,text='-',command=lambda:my_fun('minus'))
b2.grid(row=2,column=3)
my_w.mainloop()
Zoom Parent window : More about managing geometry →
rowconfigure() & columnconfigure() to Zoom widgets along with window»
« Tkinter Text
« Python Tkinter Entry How to Validate user entered data→
Managing Font option using Menu
← Subscribe to our YouTube Channel here